Advanced Options
Explore optional parameters you can use to customize and fine-tune your API requests. These settings give you greater control and flexibility depending on your use case.
Set Aspect Ratio
The aspect ratio of a video determines the width-to-height proportion of the frame. Choosing the right ratio is essential to ensure your clips look good on the platforms you’re targeting.
The ratioOfClip
parameter controls the output video shape for all generated clips. You can choose one format per request.
🔢 Parameter Values and Meanings
Parameter | Value | Aspect Ratio | Best For |
---|---|---|---|
ratioOfClip | 1 (default) | 9:16 (Vertical) | TikTok, Instagram Reels, Shorts |
2 | 1:1 (Square) | Instagram Feed, Facebook Feed | |
3 | 4:5 (Portrait) | Instagram Feed (optimized height) | |
4 | 16:9 (Horizontal) | YouTube, LinkedIn, Twitter |
Usage Examples
- ✅ ratioOfClip: 1 → Generates vertical mobile-friendly videos, ideal for TikTok or YouTube Shorts.
- ✅ ratioOfClip: 4 → Creates widescreen clips perfect for YouTube or Twitter.
- ✅ ratioOfClip: 2 → Makes square videos that maintain visibility in Instagram or Facebook feeds without cropping.
Apply a Template
The templateId
parameter allows you to apply a custom template to the generated clips. Templates can be created in the Vizard web interface that control:
- Subtitle font, size, and color
- Headline style and positioning
- Logo placement
- Branding elements
- Background layers, motion, and transitions
The template ratio must match the
ratioOfClip
parameter. Both personal templates and those in the Brand Kit are supported.
🛠️ Where to find your templateId
?
- Log in to your Vizard account.
- Open video editor by clicking on the 'Edit' button of any video clip.
- Switch to 'Template' tab.
- Hover over your desired template and copy its template ID.
- Paste it into your API request.
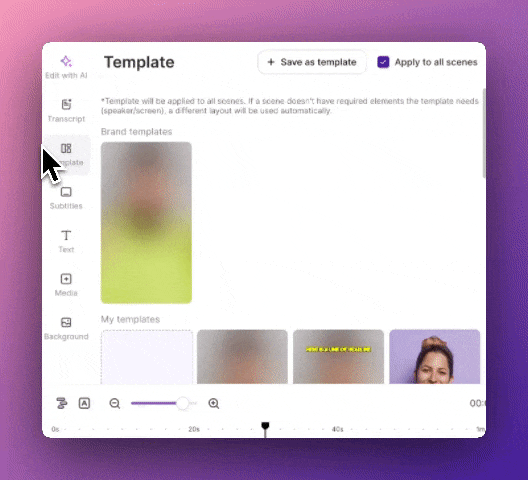
Remove Silence and Filler Words
The removeSilenceSwitch
parameter controls whether silent gaps and filler words (like “um”, “uh”) are removed from your final clips. When enabled, Vizard will automatically trim unnecessary pauses and filter filler words from the final clips for a sharper and more polished output.
This function works the same as it does in the video editor.
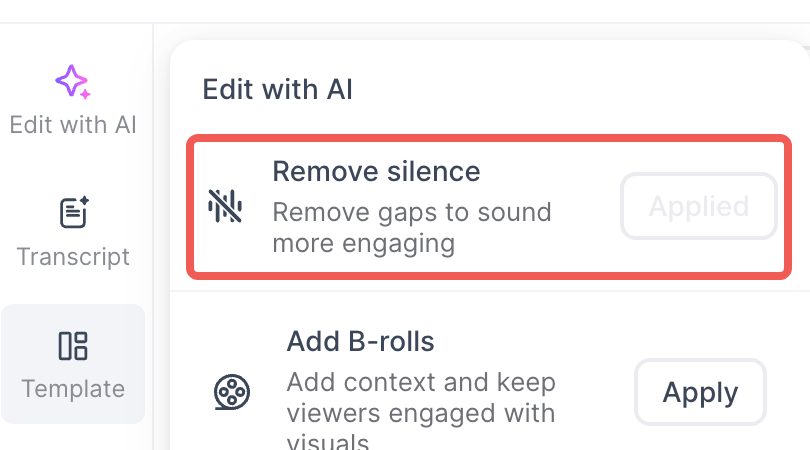
This is especially useful for:
- ✅ Interview or podcast-style videos
- ✅ Long monologues with natural pauses
- ✅ Clips meant for fast-paced social platforms
🔢 Parameter Values and Meanings
Parameter | Value | Remove silence? |
---|---|---|
removeSilenceSwitch | 0 (default) | No |
1 | Yes |
The “remove silence” option is turned off by default, as removing too many pauses can make the final video feel choppy or glitchy.
Set Maximum Number of Clips
Parameter maxClipNumber
sets the maximum number of clips to return from a long video (range: 1 to 100).
This does not control the exact number of clips—only the upper limit. If this parameter is not included in your request, all clips will be returned.
For example, if you upload a 1-hour video and the system generates 40 clips, setting maxClipNumber
to 10 will return only the top 10 clips, ranked by viral score.
Setting a smaller number can help speed up the response time.
Include Relevant Topics
Use the keywords
parameter to include content related to specific topics in your clips.
To include multiple keywords, separate them with commas.
This setting is also available in the web interface when submitting a long video.

Example:
"keywords": "AI, productivity, marketing"
Show Subtitles
Use the subtitleSwitch
parameter to control whether subtitles are displayed in the final clips.
By default, this is set to 1, meaning subtitles are turned on.
- Set to 1 → Show subtitles (default)
- Set to 0 → Hide subtitles
This setting is also available in the web interface.
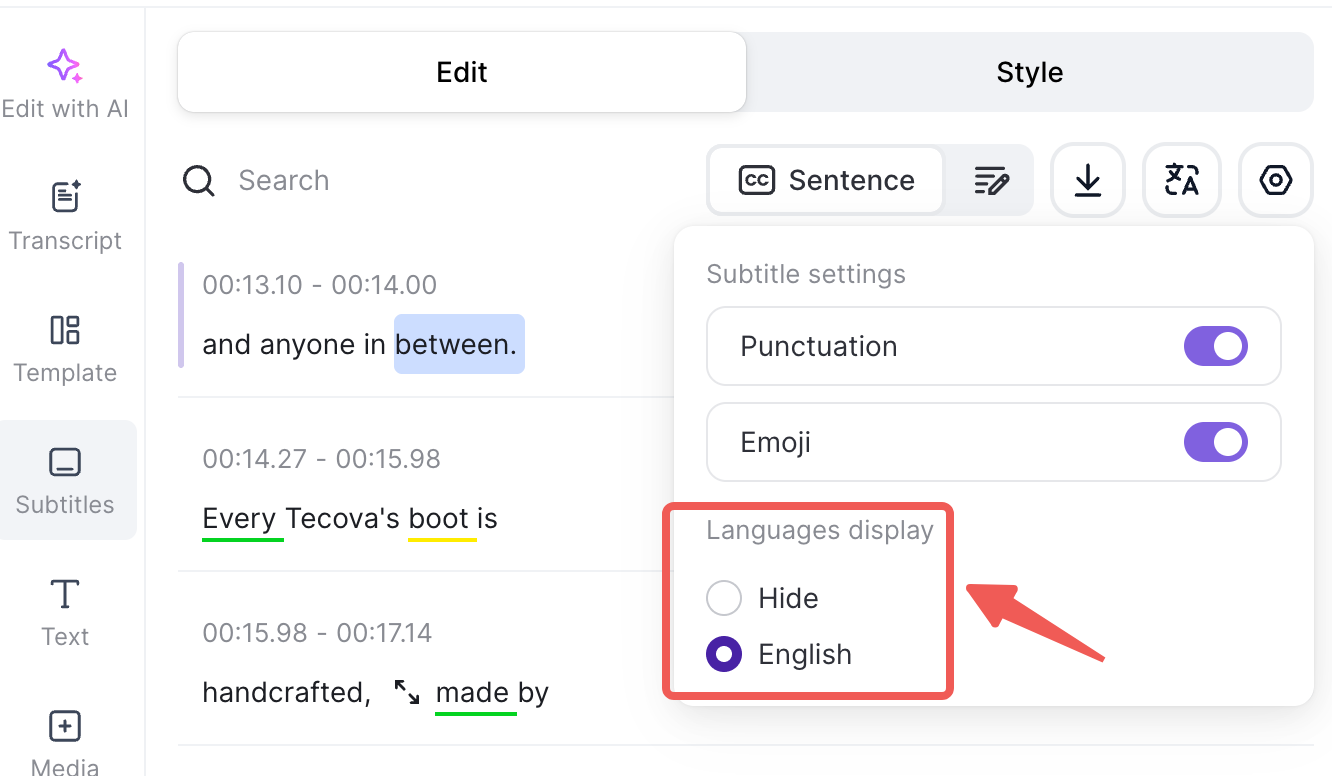
Show an AI-Generated Headline
Use the headlineSwitch
parameter to control whether an AI-generated headline (also called a hook) is added to your clip.
The headline appears as a text overlay in the first 3 seconds to grab viewers’ attention and boost engagement.
- Set to 1 → Show headline (default)
- Set to 0 → No headline
Example of a headline.
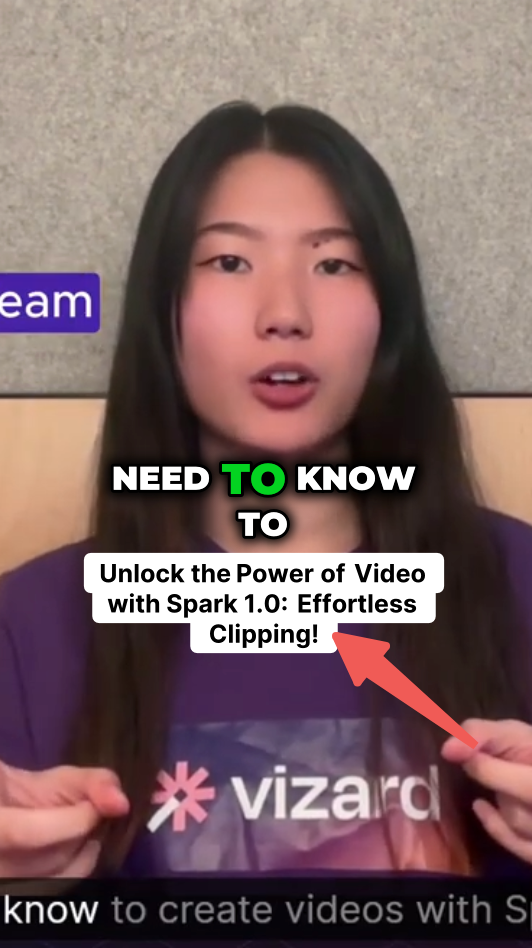
Name Your Project
Use the projectName
parameter to set a custom name for the clipping project created from your submitted video.
If not provided, the default name will be: the filename of your uploaded video, or the title of a YouTube video (if submitting via YouTube link).
Example Request
Below is an example request using some of the optional parameters introduced above. None of the parameters on this page are required—feel free to choose the ones that best fit your use case.
curl -X POST https://elb-api.vizard.ai/hvizard-server-front/open-api/v1/project/create \
-H "Content-Type: application/json" \
-H "VIZARDAI_API_KEY: YOUR_API_KEY" \
-d '{
"lang": "en",
"preferLength": [0],
"videoUrl": "https://www.youtube.com/watch?v=OqLfw-TzzfI",
"videoType": 2,
"ratioOfClip": 1,
"templateId": 52987165,
"removeSilenceSwitch": 0,
"maxClipNumber": 10,
"keyword": "AI, spark, vizard",
"subtitleSwitch": 1,
"headlineSwitch": 1,
"projectName": "Introducing Spark 1.0 - Clip any video with a simple prompt now!"
}'
import requests
url = "https://elb-api.vizard.ai/hvizard-server-front/open-api/v1/project/create"
headers = {
"Content-Type": "application/json",
"VIZARDAI_API_KEY": "YOUR_API_KEY"
}
data = {
"lang": "en",
"preferLength": [0],
"videoUrl": "https://www.youtube.com/watch?v=OqLfw-TzzfI",
"videoType": 2,
"ratioOfClip": 1,
"templateId": 52987165,
"removeSilenceSwitch": 0,
"maxClipNumber": 10,
"keyword": "AI, spark, vizard",
"subtitleSwitch": 1,
"headlineSwitch": 1,
"projectName": "Introducing Spark 1.0 - Clip any video with a simple prompt now!"
}
response = requests.post(url, headers=headers, json=data)
print(response.status_code)
print(response.json())
import axios from 'axios';
const url = 'https://elb-api.vizard.ai/hvizard-server-front/open-api/v1/project/create';
const headers = {
'Content-Type': 'application/json',
'VIZARDAI_API_KEY': 'YOUR_API_KEY'
};
const data = {
lang: 'en',
preferLength: [0],
videoUrl: 'https://www.youtube.com/watch?v=OqLfw-TzzfI',
videoType: 2,
ratioOfClip: 1,
templateId: 52987165,
removeSilenceSwitch: 0,
maxClipNumber: 10,
keyword: 'AI, spark, vizard',
subtitleSwitch: 1,
headlineSwitch: 1,
projectName: 'Introducing Spark 1.0 - Clip any video with a simple prompt now!'
};
axios.post(url, data, { headers })
.then(res => {
console.log(res.status);
console.log(res.data);
})
.catch(err => {
console.error(err.response?.data || err.message);
});
Updated 3 months ago